Trong bài viết lần này, chúng ta hãy cùng tìm hiểu 1 hook mới trong ReactJS là useMemo và 1 HOC trong ReactJS là memo nhé!😄
Trong quá trình làm việc với React, chúng ta sẽ có lựa chọn như:
- Hooks
- render props
- higher-order-components (HOCs)
Mình sẽ nói cụ thể hơn 3 phần này khác gì nhau ở bài viết sau nhé 😄
1. memo trong ReactJS
mình có 1 đoạn code đơn giản như sau
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
|
import {useState} from "react";
import {useEffect} from "react";
import Content from "./Content";
import Chat_content from "./chat_fake/Chat_content";
import UseRef from "./use_ref/UseRef";
import Memo_content from "./memo/Memo_content";
function App() {
const [show, setShow] = useState(false)
const [number, setNumber] = useState(1)
const handleSetnumber = () => {
setNumber(number+1)
}
return (
<div style={{padding: 32}}>
<div>
<Memo_content/>
</div>
<div>
{number}
</div>
<button onClick={handleSetnumber}>
Click
</button>
</div>
)
}
export default App;
|
Và đây là code của component Memo_content
1
2
3
4
5
6
7
8
9
10
11
12
|
import {memo, useEffect, useState} from "react";
function Memo_content() {
console.log('render')
return (
<div>
Hello
</div>
)
}
export default Memo_content;
|
Chúng ta có thể thấy, mỗi khi click đẩ thay đổi state của component cha thì component con là Memo_content cũng được
render lại mặc dù component con không có gì thay đổi. Vậy chúng ta sẽ xử lý vấn đề này như thế nào?
Rất đơn giản, bạn chỉ cần thêm memo để wrap lại component con như sau:
1
|
export default memo(Memo_content);
|
Thông thường với những component nặng về xử lý logic như biểu đồ, dashboard mà chúng ta không muốn render lại
component thì nên dùng cách này, còn thông thường việc này cũng không quá quan trọng 😄 theo mình là như vậy
2. useMemo trong ReactJS
Vậy khi nào chúng ta sử dụng useMemo? ĐÓ là khi chúng ta không muốn thực hiện lại 1 logic nào đó không cần thiết.
Vậy khi nào là không cần thiết? Chúng ta hãy cùng tìm hiểu qua 1 ví dụ đơn giản mình đưa ra ở dưới đây nhé!
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
|
import {useState} from "react";
function App() {
const [name, setName] = useState('')
const [price, setPrice] = useState('')
const [products, setProducts] = useState([])
const handleSubmit = () => {
setProducts([...products,{
name,
price: +price
}])
}
const total = products.reduce((result, pro) =>
{
console.log('bi tinh toan lai')
return result + pro.price
},0
)
return (
<div style={{
padding: '10px 200px'
}}>
<input
value={name}
placeholder='Enter name...'
onChange={event => setName(event.target.value)}
/>
<br/>
<br/>
<input
value={price}
placeholder='Enter price...'
onChange={event => setPrice(event.target.value)}
/>
<br/>
<br/>
<button onClick={handleSubmit}
>Add</button>
<br/>
<br/>
Total : {total}
<ul>
{
products.map((product, index) => (
<li key={index}>{product.name} - {product.price}</li>
))
}
</ul>
</div>
)
}
export default App;
|
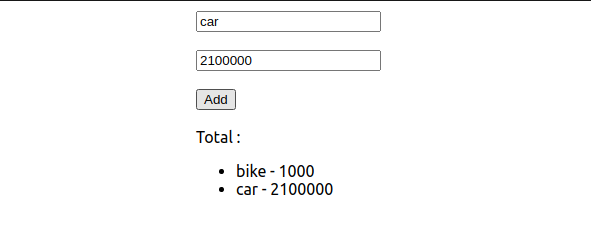
Về cơ bản ứng dụng đơn giản là như vậy😄
Tuy nhiên nếu như bạn để ý, hàm tính toán ra price của mình luôn bị gọi khi chúng ta nhập
giá trị vào ô price, mặc dù khi đó chúng ta chưa cần tính lại total. Và đây là
lúc chúng ta sử dụng useMemo như sau:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
|
import {useState, useMemo} from "react";
function App() {
const [name, setName] = useState('')
const [price, setPrice] = useState('')
const [products, setProducts] = useState([])
const handleSubmit = () => {
setProducts([...products,{
name,
price: +price
}])
}
const total = useMemo(() => {
const total = products.reduce((result, pro) =>
{
console.log('bi tinh toan lai')
return result + pro.price
},0
)
},[products])
return (
<div style={{
padding: '10px 200px'
}}>
<input
value={name}
placeholder='Enter name...'
onChange={event => setName(event.target.value)}
/>
<br/>
<br/>
<input
value={price}
placeholder='Enter price...'
onChange={event => setPrice(event.target.value)}
/>
<br/>
<br/>
<button onClick={handleSubmit}
>Add</button>
<br/>
<br/>
Total : {total}
<ul>
{
products.map((product, index) => (
<li key={index}>{product.name} - {product.price}</li>
))
}
</ul>
</div>
)
}
export default App;
|
Tương tự như useEffect và useCallback, useMemo cũng nhận 2 đối số,
đối số thứ nhất là 1 callback, đối số thứ 2 là 1 dependencies, nguyên tắc hoạt động
của dependencies cũng tương tự như useEffect và useCallback.
Hy vọng bài viết sẽ giúp ích cho bạn 😀, hẹn gặp lại mọi người ở những bài viết sau.